Stylish Sliding View Transitions with Rails 8
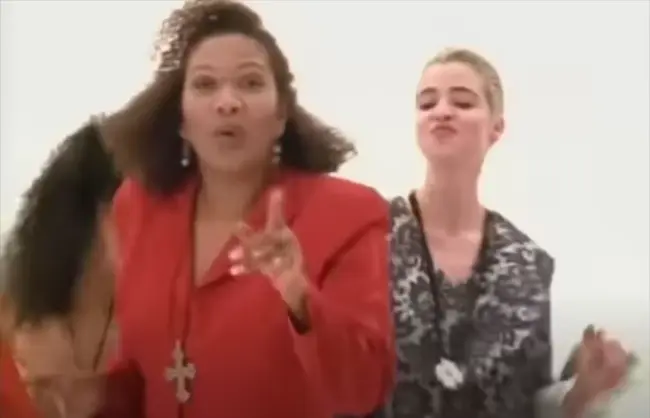
With the release of Ruby on Rails 8 and its emphasis on Progressive Web Apps (PWAs), I’ve become curious how simple it is to build a web app that’s indistinguishable from a native app. Between Hotwire, a new tweak to Turbo which directly supports the new View Transition API, and a little bit of custom code, adding killer page transitions to our web app is straightforward and is a major distinction in making our web apps feel more native.
The desired effect
Many native apps use a sliding effect to transition between views. This distinguishes information hierarchy. If you tap a particular item, its details slide in from the right, along with the back button. If you tap the back button, the details slide back to the left. If you tap to see more details, the details slide in from the right again with the back button staying in place. Go ahead and try one of the major mobile apps on your phone, and you’ll see some variation of this.
I paid particular attention to the Spotify and Nintendo Music apps, because the PWA I’m building has similarities to these. When you’re poking around in albums, the sliding effect is very useful in conveying that you’re navigating deeper into the app. If you hadn’t noticed before, you probably won’t unsee it!
We’ll apply this effect to our PWA. We’ll navigate to “My Profile”, which should trigger the sliding effect with a back arrow. We’ll click into a subsection of My Profile, which should slide in the new content, but not the back arrow. We’ll then go back and see the same slide behaviors happen in reverse.
The View Transition API
The View Transition API is relatively new, but is supported by most major browsers today. It can be implemented in multi-page app (MPA) or single-page app (SPA) flavors. Turbo supports the SPA implementation, which is activated via document.startViewTransition
.
You can call this function in any web project. Within its callback, you would make changes to the DOM that represent the “new” view. The View Transition API will then “capture” the state of the old view and apply an “old” pseudo-class which is animated. The new view will have a “new” pseudo-class applied to it, which is also animated.
Animations are declared via CSS selectors, some made available by the View Transition API. New properties are also made available with the View Transition API.
The Hotwire way
Turning on the View Transition API for Turbo requires adding a single meta tag to your application:
<meta name="view-transition" content="same-origin" />
And voila, you’re using view transitions powered by Turbo. As you navigate the site, you should see a cross fade effect. This works because Turbo is fetching new HTML content on navigation, and updating the DOM within document.startViewTransition
. Importantly, the animation is blocked on Turbo returning the next document.
If you look closely in the inspector during a transition, you’ll see the html
element flicker with a data-turbo-visit-direction
attribute, which is an added bonus for contextualizing if the user navigated forward or backward. We’ll be using this later to help differentiate sliding behaviors (left or right)
While a cross-fade is a nice effect, it’s not what we’re looking for. Let’s start writing some CSS.
Using CSS to modify the transition
When you opt into view transitions, you get a free root
animation group. Everything inherits from this root, but they can be overridden. The default root animation is the cross fade we are seeing.
Let’s apply a sliding effect to all of the page transitions:
@keyframes slide-in-from-right {
from {
transform: translateX(100%);
}
}
&::view-transition-new(root) {
animation-name: slide-in-from-right;
}
Now if you transition between pages, the cross-fade is replaced with a slide-in effect. If you pay close attention, you will still see the “old” content fade as the new content slides in. That’s because we only applied the slide-in effect to the “new” pseudo-selector.
If we wanted the old content to slide out instead, we can animate it separately:
@keyframes slide-out-to-left {
to {
transform: translateX(-100%);
}
}
&::view-transition-old(root) {
animation-name: slide-out-to-left;
}
You should now see the “old” content slide away to the left instead of fading out.
Now we have a pretty nice slide effect! But we still have a couple of problems. The first is that when you click the back button, the new content still slides in from the right, but we want the opposite to happen.
Turbo to the rescue! The free data-turbo-visit-direction
attribute it adds to the DOM helps us solve this exact problem:
@keyframes slide-in-from-left {
from {
transform: translateX(-100%);
}
}
@keyframes slide-out-to-right {
to {
transform: translateX(100%);
}
}
html[data-turbo-visit-direction="back"] {
&::view-transition-old(root) {
animation-name: slide-out-to-right;
}
&::view-transition-new(root) {
animation-name: slide-in-from-left;
}
}
html[data-turbo-visit-direction="forward"] {
&::view-transition-old(root) {
animation-name: slide-out-to-left;
}
&::view-transition-new(root) {
animation-name: slide-in-from-right;
}
}
And now when we navigate back, the content should slide in the opposite direction. Note that Rails’s link_to :back
may not always provide the desired effect since it may render a link to a new page. So consider writing your own history.back()
handler instead.
The second problem is that the footer and back button always animate, even if their placement will not change, which breaks the hierarchical context. If the back button is visible on both the “old” and “new” views, it should not animate. Since the footer never changes, it should never animate.
We’ll need to separate the back button from the root
animation. If you don’t want animations to be applied to all elements, you’ll need to break them out into their own view transitions.
Let’s assume the back button is inside a header
and middle content is in main
. Both of these will selectively slide depending on how we’re navigating the app. The footer
should never slide.
/* All animation groups will have a duration of 600ms */
::view-transition-group(*) {
animation-duration: 600ms;
}
/* ... except for the root animation, which will have animations disabled.
This is for the footer */
::view-transition-group(root) {
animation-duration: 0ms;
}
/* The main content gets its own view transition instead of root. The name
is user-defined, and we'll reference it later */
main {
view-transition-name: mainSlide;
}
/* Same for the header */
header {
view-transition-name: headerSlide;
}
/* Apply the animation to these view transitions by name, instead of to root */
html[data-turbo-visit-direction="back"] {
&::view-transition-old(mainSlide) {
animation-name: slide-out-to-right;
}
&::view-transition-new(mainSlide) {
animation-name: slide-in-from-left;
}
&::view-transition-old(headerSlide) {
animation-name: slide-out-to-right;
}
&::view-transition-new(headerSlide) {
animation-name: slide-in-from-left;
}
}
html[data-turbo-visit-direction="forward"] {
&::view-transition-old(mainSlide) {
animation-name: slide-out-to-left;
}
&::view-transition-new(mainSlide) {
animation-name: slide-in-from-right;
}
&::view-transition-old(headerSlide) {
animation-name: slide-out-to-left;
}
&::view-transition-new(headerSlide) {
animation-name: slide-in-from-right;
}
}
By breaking out the main
and header
elements into their own view transitions, we can selectively apply animations to them. The view-transition-name
property cannot be applied to more than one element, though, so we may need to define similar behaviors more than once.
Notice that the footer
no longer slides at all.
Now we need to differentiate behaviors between header
and main
. Since we use CSS to declare view transition names, we might naturally reach for rendering different properties directly onto header
or main
from the server. I tried this approach at first, and ran into these problems:
First, contextualizing navigation on the server increased the complexity of both my controllers and views, and felt difficult to maintain, particularly for something cosmetic.
Second, Turbo caches “previews” of pages for smoother rendering. Server-rendered classes may swap out once the cache is busted. This can cause class names to change on the DOM before the view transition animates, leading to animation bugs. While you can disable this cache, you’d be missing out on a very nice feature of Turbo that also makes your PWA feel more like a native app.
Taking some inspiration from Turbo’s own data-turbo-visit-direction
trick, we can implement something similar. If you can contextualize the entire user navigation at the root of the DOM, writing the transitions becomes much simpler.
Let’s implement a Stimulus controller which will “contextualize” the DOM based on how the user is navigating.
import {Controller} from "@hotwired/stimulus"
export default class extends Controller {
disconnect() {
window.removeEventListener("turbo:visit", this.#handleTurboVisit);
}
connect() {
/*
Store the current path. You can also try to read this in an event handler,
but I found this to be prone to race conditions depending on browser and
if the back button is used.
The connect() lifecycle hook is pretty consistent as long as it's connected
to the body
*/
this.beforePath = window.location.pathname;
/*
The transition context will be attached to the html tag, just like
data-turbo-visit-direction. We do break Stimulus encapsulation here, but we
can safely assume the document will have the html tag.
*/
this.html = document.querySelector("html");
/*
As long as Turbo is on, this event should fire on every navigation, including
the back button
*/
window.addEventListener("turbo:visit", this.#handleTurboVisit);
}
/*
Simple regex to check if path is a profile page or a child of the profile page.
This approach depends on structured routing vernacular. If you have complicated
routing business logic, consider using embedded Ruby values to be read
by Stimulus
*/
#isProfilePage(path) {
return (path.match("user_profile"))
}
/*
Declarative methods for checking the nature of a transition. In this case, is
the prior page or the new page within the profile domain? This case is
important since the header would need to slide
*/
#isNavigatingToOrFromProfile(newPath) {
return((
this.#isProfilePage(newPath) && !this.#isProfilePage(this.beforePath)
) || (
!this.#isProfilePage(newPath) && this.#isProfilePage(this.beforePath)
));
}
/*
Same as above, but for checking if we're navigating between individual
profile pages. In this case, the header doesn't slide, but main does
*/
#isNavigatingBetweenProfileDetails(newPath) {
return(this.#isProfilePage(newPath) || this.#isProfilePage(this.beforePath));
}
/*
Evaluate all the transition "rules" and add them to the html tag as
data attributes
*/
#updateTemplate(newPath) {
const transitionContexts = [];
if (this.#isNavigatingToOrFromProfile(newPath)) {
transitionContexts.push("navigatingBetweenDetailAndList");
}
if (this.#isNavigatingBetweenProfileDetails(newPath)) {
transitionContexts.push("navigatingDetails");
}
if(transitionContexts.length > 0) {
this.html.dataset.transitionContext = transitionContexts.join(" ");
} else {
delete this.html.dataset.transitionContext;
}
}
#handleTurboVisit = (event) => {
this.#updateTemplate(event.detail.url);
}
}
Finally, use this data attribute for the transition CSS code:
html[data-transition-context~="navigatingDetails"] main {
view-transition-name: mainSlide;
}
html[data-transition-context~="navigatingBetweenDetailAndList"] header {
view-transition-name: headerSlide;
}
Now if we move around the app, we’ll see the slide behavior only triggers when we visit the user profile, and the back button will not animate unless we leave the profile context:
Now that looks nice! We can adapt this approach to other types of navigation, and even break out sub-elements to animate in or out independently depending on the navigation. This is a nice way to introduce animation behaviors without adding a bunch of statefulness and complexity to your Ruby code.